BASIC Grayscale Using xLIB
Note: This tutorial requires some calculator files to be downloaded and transferred to your calculator for examples. You can
find all the files in this zipped file. Please download before continuing.
This is a tutorial on using xLIB and BASIC to create 3 and 4 level grayscale on your 83+/SE or 84+/SE calculator. First off, you need xLIB (included) and some free time. Send xLIB.8xk to your calc, then press [APPS] then select “xLIB” then press [ENTER] then [1] then [3]. Now xLIB is installed, here’s the most important part, what kind of sprites are you going to make? 3lv GS sprites are by far the easiest, smallest, and most common. 4lv GS sprites are a bit harder, but look better, and come in 2 flavors. We’ll wait while you decide… Okay, done yet? Good, there’s below are the sections for what kind of sprite you want to make! Remember, the most important thing in making a game isn’t what OTHER people want, but what YOU want!
-=3lv GS Sprites=-
These are by far the easiest to make, and use, they are also the smallest when it comes to pic size. In order to make these you’ll need to have an image in mind, I’ve supplied one for ease of use.

This, however, is a finished sprite. You need 2 sprites in reality to make this. You need a base

and a mask

. These combined give you a finished sprite. In order to get a base and mask you take your finished sprite

and put a blackened pixel “pattern” over the grey areas,

then clear out all the grey

to get your base. The mask is much simpler; all you need to do for it is color all the black in the finished sprite white, and color all the grey in the finished sprite black to get

. Simple right? Good, now you need to write the code to get the grey. Since you’re using xLIB you have 255 pics and can use pic 255 for a “mask pic”. Put your base in the top left of PIC0 and your mask in the top left of PIC1. Now make a new program, use the code below to do this.
prgmGS - Name of the program
:real(0:real(5,0,0 - Clears the screen, sets the contrast to 0
:DelVar [A]{8,8->dim([A] - Deletes [A] and then sets it’s dimensions to 8 by 8
:real(2,0,0,0,8,8,0,8,0,8,1 - Draws a tilemap from PIC1
:real(9,1,255:real(9,0,255 - Deletes PIC255 then stores PIC255
:real(2,0,0,0,8,8,0,8,0,8,0 - Draws a tilemap from PIC0
:real(5,1:real(5,0,Ans - Get’s the real contrast, sets it to that
:Repeat getKey - Repeat until a key is pressed
:real(3,255,3 - xor PIC255
:End:real(9,1,255 - Ends the repeat loop, delete PIC255
Okay, now run the program. You should get (had you installed xLIB) the entire screen tall, by 2/3 of the screen wide filled with

to be more exact, 64 of them. Now, you say you want to be able to move things around? This is where it gets tricky, you can move things around, but you need to remember to put :real(3,255,3 about every 2-3 lines to keep the GS whilst you move. This is (in my honest opinion) everything you need to make beautiful 3 level grayscale sprites! Have fun!
-=4lv GS Overwrite Sprites=-
WARNING! This method is NOT for game play use, only for still pictures like title screens or just to show off. DO NOT USE THIS FOR GAME PLAY!
Okay, now that that’s out of the way we get to the sprite making. 4lv GS sprites are much more complicated than 3lv GS ones, but give a better end result, more grays. Here’s an example

looks a lot better than out 3lv one right? Well this in reality is 3 sprites, frame 1

, frame 2

, and frame 3

. If it’s a bit hard to see what I’ve done here (and it is) I’ve also included a chart for this. Basically, the grays for the first and third frames are the same. Basically the main difference between light and dark grey here is frame 2, light grey in frame 2 is white, and dark grey is black. For that split second your eye registers the white and black and makes out 2 different shades of grey.

This should help a bit. I’ve also included the 3 frames to make

on your calculator (this is one of the trees that won Omnmaga Pixel Art Contest 3) so you can see another example of a larger sprite. The method for making the sprites is exactly the same as 3lv GS but you need to do it 2 times, and have opposite patterns, and of course frame 2. Okay, take the frame 1 sprite (above) and store it into the top left of PIC0, frame 3 goes in the top left of PIC1, and frame 3 goes in the top left of PIC2. Okay, then you need to run this code.
prgmGS2
:real(0:real(5,0,0
:DelVar [A]{8,8->dim([A]
:real(2,0,0,0,8,8,0,8,0,8,0
:real(9,1,253:real(9,0,253
:real(2,0,0,0,8,8,0,8,0,8,1
:real(9,1,254:real(9,0,254
:real(2,0,0,0,8,8,0,8,0,8,2
:real(9,1,255:real(9,0,255
:real(5,1,0:real(5,0,Ans
:Repeat getKey
:real(3,253:real(3,254
:real(3,255:End
:real(9,1,255:real(9,1,254
:real(9,1,253
Okay now run the program, (there are no comments because it’s the same function as the other one) you should get a similar result as with the 3lv program, the difference here is a) 4 levels of grey; and b) on the 83+ you’ll notice an oddity with how dark/light the grays are (that’s due to the slower calc). Now, since this isn’t to be used for normal game play you don’t need anything about walking engines or sorts. :) This is a nice little bit for title screens in BASIC or to brag that you can. (but don’t brag)
-=4lv XOR Sprites=-
Here’s the section about 4lv GS sprites for game play use. These are a bit bigger (4 actual sprites) but produce an effect you can use in a game. The end product will be the same sprite

but the frames will be different. Frame 1 will be a 1 display only frame and will look exactly like frame 1 of the 4lv overwrite sprite

. Now is where the difference shows, you need to get an end result of

by xoring frame 2 over frame 1, thus, our frame 2 will look like

. Okay, now we need to get from

to

by xoring another frame over our sprites. Thusly our frame 3 will look something like

, this looks similar to frame 2, but trust me, it isn’t. Okay, now that we have

we need to get back to

. This is the simplest frame. Frame 4 looks like .

BAM! You’ve got a 4lv GS sprite that you can use in a game! If you’re not seeing how I did that I have yet another chart :o to help you!
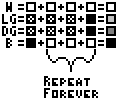
Granted it DOES take a few more steps and a few more sprites the end result is worth it. Okay, now the fun part (because making GS sprites isn’t?!?!?) store frame 1 into the top left of PIC0, frame 2 into PIC1, frame 3 into PIC2, and frame 4 into PIC3. The run this.
prgmGS3
:real(0:real(5,0,0
:DelVar [A]{8,8->dim([A]
:real(2,0,0,0,8,8,0,8,0,8,3
:real(9,1,255:real(9,0,255
:real(2,0,0,0,8,8,0,8,0,8,2
:real(9,1,254:real(9,0,254
:real(2,0,0,0,8,8,0,8,0,8,1
:real(9,1,253:real(9,0,253
:real(2,0,0,0,8,8,0,8,0,8,0
:real(5,1,0:real(5,0,Ans
:Repeat getKey
:real(3,253,3:real(3,254,3
:real(3,255,3:End
:real(9,1,255:real(9,1,254
:real(9,1,253
Okay, now run this program, you should get the exact same thing as with the 4lv overwrite program, but you can also have other things about and have them not be cleared :) This is good for game play. Pretty huh? Good, you probably should exit the program before you kill your batteries. I’ve also included the pics in order to make the GS tree (see last section) using this method. As for using in game play, well use the same method you’d use for 3lv GS, but use the 3 real(3 commands in order (253, 254, then 255) and you should be good to go! Remember though, this method is NOT recommended for the normal 83+ as it produces a weird effect.
-=Conclusion=-
Well go on, make your sprites! Have fun! And make games you like! Thanks for reading this and considering my methods. If you glance at the folder/zip that this tut comes in you’ll notice a few things, .8xi files, an .8xp file, and a few other images. The .8xi and .8xp files are to be sent to your calc so you can see a 4lv GS tree example. The .png files are visual examples of the .8xi files, and the XORPIC#.png files are examples of the same tree, using the 4lv xor method. Good luck!
-Fred Sparks (CDI)